Getting Started With React Hooks By Example: UseState, UseEffect
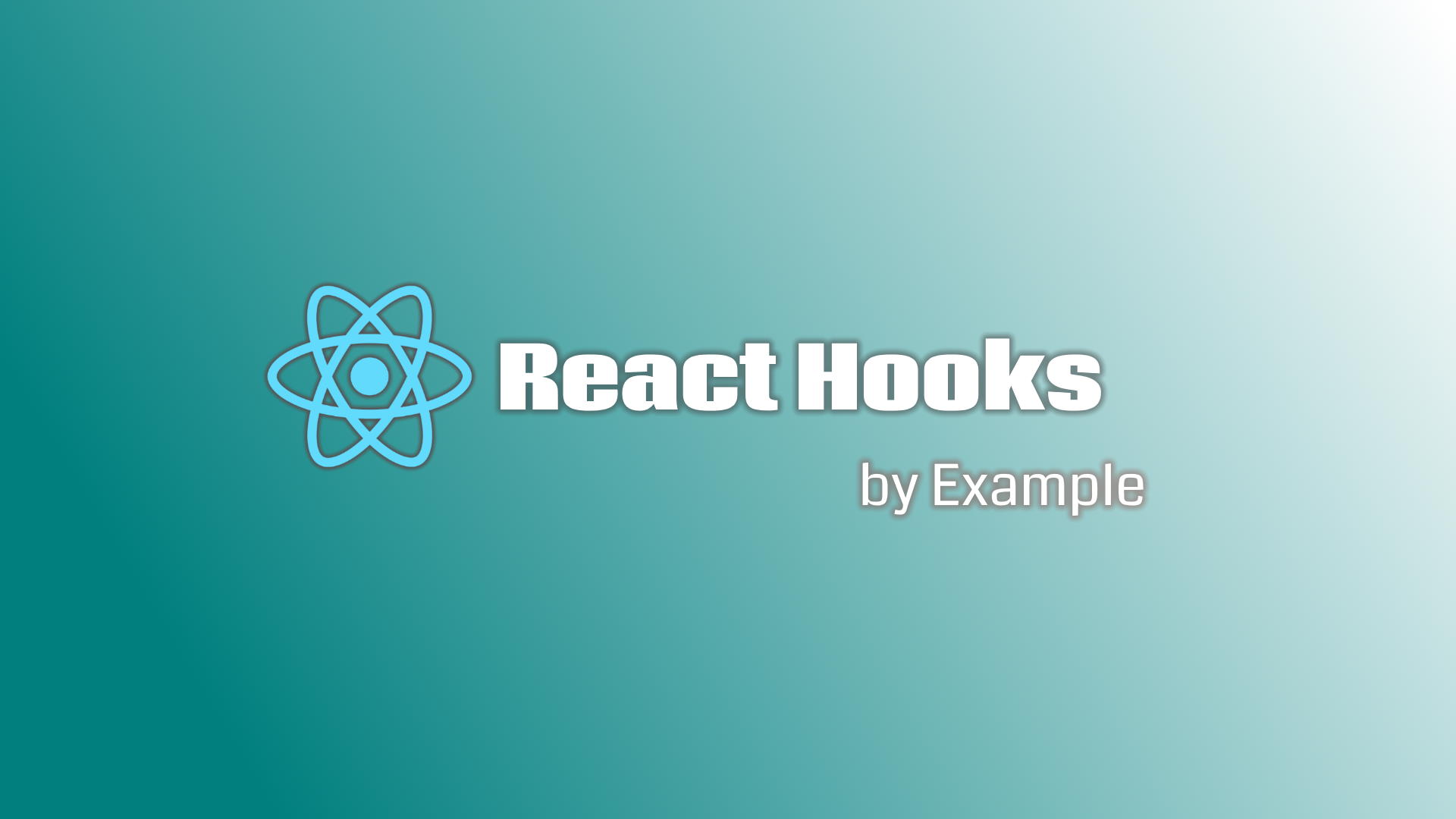
Get introduced to React Hooks, useState and useEffect to write less complex components and better reuse stateful behavior.
Introduction
Hooks were introduced starting from the 16.8 release of React, the open-source front-end library made by Facebook. They allow function components to "hook into" React state and lifecycle features without having to write classes.
The main motivations behind React Hooks are:
- Provide a better way to share stateful logic between components using Custom Hooks, as an alternative to render props and higher-order components.
- Reduce the complexity of components by allowing a better separation of concerns for side-effects using Effect Hooks, as an alternative to lifecycle methods. As a result, the component tree's structure is more flat because less wrapper components are needed.
Hooks are also supported by React Native starting from the 0.59 version.
Adding state with useState
import React, { useState } from 'react';
function StateExample() {
const [visible, setVisible] = useState(true);
return (
<div>
<button onClick={() => setVisible(!visible)}>
Toggle visibility
</button>
{ visible ? <p>I am visible!</p> : null }
</div>
);
}
useState
, also called the State Hook, is used to introduce local state to function components:
- It accepts the initial state value as an argument.
- It returns a pair: the current state value and a function that lets you update it.
You can have multiple State Hooks in the same component:
Performing side-effects with useEffect
import React, { useState, useEffect } from 'react';
function EffectExample() {
const [name, setName] = useState("");
const [displayText, setDisplayText] = useState("");
useEffect(() => {
const interval = setInterval(() => {
const time = new Date().toLocaleTimeString();
setDisplayText(`Hello ${name} ! The current time is: ${time}`)
}, 1000);
return () => clearInterval(interval);
}, [name]);
return (
<div>
<input
type="text"
value={name}
onChange={ e => setName(e.target.value) }
placeholder="Name..." />
<p>{displayText}</p>
</div>
);
}
useEffect
, aka the Effect Hook, lets you perform side effects in function components (such as requesting data, subscribing to events, manual DOM mutations, logging, ...):
- It accepts as the first argument a function that will run after each render. This effect function can optionally return a clean-up function to cancel subscriptions and free up other used resources.
- An optional second argument can be added to conditionally fire the effect: the array of values that the effect depends on: the values referenced inside the effect function. If this array is empty, the effect will be run only once.